튜토리얼
.NET 개발환경에서 팝빌 SDK를 추가하여 전자명세서 즉시 발행 (RegistIssue) 함수를 구현하는 예시입니다.
1. POPBiLL SDK 추가
① 팝빌 연동자료실에서 ASP.NET SDK 예제코드 다운로드 후 압축을 해제합니다.
② 다운받은 SDK 예제코드의 Linkhub/, Popbill/ 폴더를 SDK를 적용할 프로젝트 경로에 복사하고. Linkhub.csproj, Popbill.csproj를 각각 기존 프로젝트로 추가합니다.
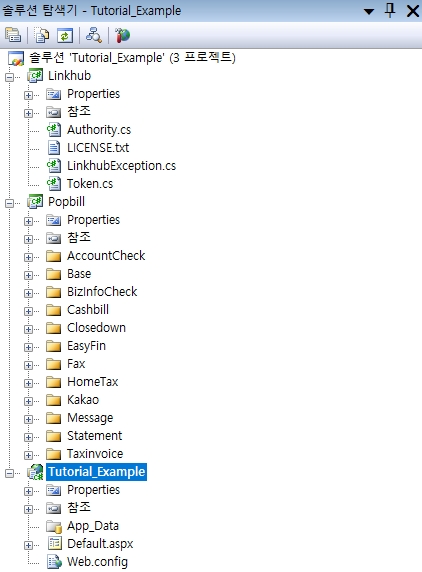
③ Popbill 프로젝트를 적용할 프로젝트의 참조로 추가합니다.
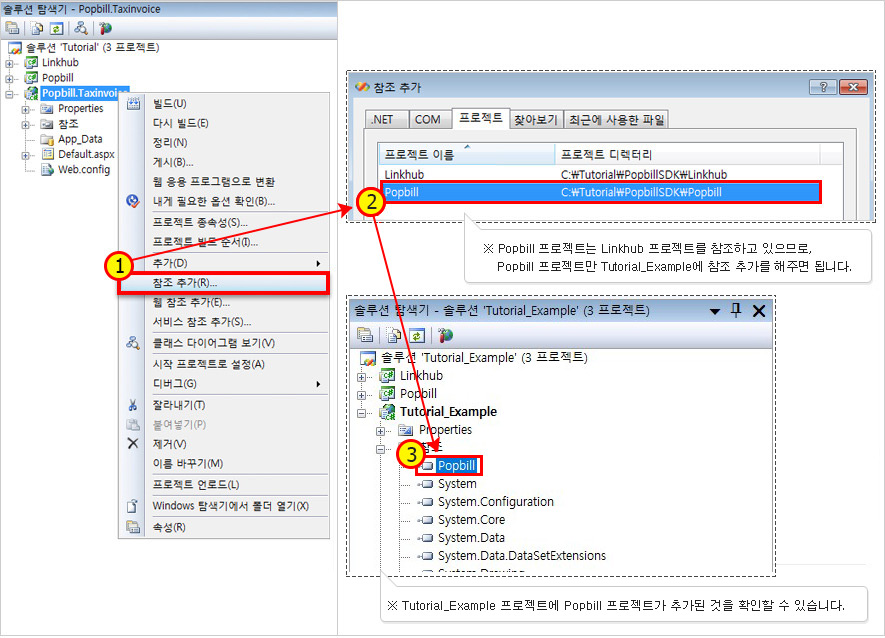
2. POPBiLL SDK 설정
프로젝트에 Global.asax 파일을 생성하여 연동환경 설정값, 전자명세서 서비스 클래스를 선언하고 Application_Start() 함수에 전자명세서 서비스 클래스 초기화 코드를 추가합니다.
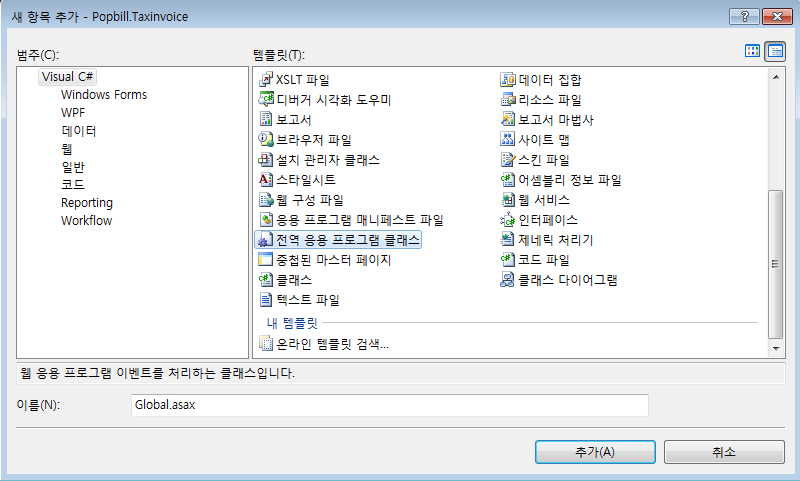
using System;
using System.Collections;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.SessionState;
using System.Xml.Linq;
using Popbill.Statement;
namespace Tutorial_Example
{
public class Global : System.Web.HttpApplication
{
// 링크아이디
private string LinkID = "TESTER";
// 비밀키
private string SecretKey = "SwWxqU+0TExEXy/9TVjKPExI2VTUMMSLZtJf3Ed8q3I=";
// 전자명세서 서비스 객체 선언
public static StatementService statementService;
protected void Application_Start(object sender, EventArgs e)
{
// 전자명세서 서비스 객체 초기화
statementService = new StatementService(LinkID, SecretKey);
// 연동환경 설정, true-테스트, false-운영(Production), (기본값:false)
statementService.IsTest = true;
// 인증토큰 IP 검증 설정, true-사용, false-미사용, (기본값:true)
statementService.IPRestrictOnOff = true;
// 통신 IP 고정, true-사용, false-미사용, (기본값:false)
statementService.UseStaticIP = false;
// 로컬시스템 시간 사용여부, true-사용, false-미사용, (기본값:false)
statementService.UseLocalTimeYN = true;
}
}
}
3. RegistIssue 기능 구현
Web Form을 추가하여 registIssue.aspx를 생성하여 응답코드, 메시지 확인 페이지를 추가하고, registIssue.aspx.cs 파일의 Page_Load 이벤트에 함수 호출 코드를 추가합니다.
registIssue.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="registIssue.aspx.cs" Inherits="Popbill.Statement.Example.registIssue" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Popbill Statement</title>
</head>
<body>
<div>
<p>Response</p>
<br/>
<fieldset>
<legend>전자명세서 즉시 발행</legend>
<ul>
<li>Response.code : <%= code %></li>
<li>Response.message : <%= message %></li>
</ul>
</fieldset>
</div>
</body>
</html>
registIssue.aspx.cs
using System;
using System.Collections;
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
namespace Popbill.Statement.Example
{
public partial class registIssue : System.Web.UI.Page
{
public String code;
public String message;
protected void Page_Load(object sender, EventArgs e)
{
// 팝빌회원 사업자번호, '-' 제외 10자리
String testCorpNum = "1234567890";
// 팝빌회원 아이디
String testUserID = "testkorea";
// 맞춤양식코드, 미기재시 기본양식으로 처리
String formCode = "";
// 명세서 종류 코드 - 121(거래명세서), 122(청구서), 123(견적서), 124(발주서), 125(입금표), 126(영수증)
int itemCode = 121;
// 전자명세서 문서번호
String mgtKey = "20250314-001";
// 즉시 발행 메모
String memo = "즉시 발행 메모";
// 전자명세서 객체
Statement statement = new Statement();
// [필수], 기재상 작성일자 날짜형식(yyyyMMdd)
statement.writeDate = "20250314";
// [필수], {영수, 청구, 없음} 중 기재
statement.purposeType = "영수";
// [필수], 과세형태, {과세, 영세, 면세} 중 기재
statement.taxType = "과세";
// 맞춤양식코드, 기본값을 공백('')으로 처리하면 기본양식으로 처리.
statement.formCode = formCode;
// [필수] 전자명세서 양식코드
statement.itemCode = itemCode;
// [필수] 문서번호, 1~24자리 숫자, 영문, '-', '_' 조합으로 사업자별로 중복되지 않도록 구성
statement.mgtKey = mgtKey;
/**************************************************************************
* 발신자 정보 *
**************************************************************************/
// [필수] 발신자 사업자번호
statement.senderCorpNum = testCorpNum;
// 종사업장 식별번호. 필요시 기재. 형식은 숫자 4자리.
statement.senderTaxRegID = "";
// 발신자 상호
statement.senderCorpName = "발신자 상호";
// 발신자 대표자 성명
statement.senderCEOName = "발신자 대표자 성명";
// 발신자 주소
statement.senderAddr = "발신자 주소";
// 발신자 종목
statement.senderBizClass = "발신자 종목";
// 발신자 업태
statement.senderBizType = "발신자 업태,업태2";
// 발신자 담당자 성명
statement.senderContactName = "발신자 담당자명";
// 발신자 메일주소
statement.senderEmail = "test@test.com";
// 발신자 연락처
statement.senderTEL = "070-7070-0707";
// 발신자 휴대폰번호
statement.senderHP = "010-000-2222";
/**************************************************************************
* 수신자 정보 *
**************************************************************************/
// 수신자 사업자번호
statement.receiverCorpNum = "8888888888";
// [필수] 수신자 상호
statement.receiverCorpName = "수신자 상호";
// 수신자 대표자 성명
statement.receiverCEOName = "수신자 대표자 성명";
// 수신자 주소
statement.receiverAddr = "수신자 주소";
// 수신자 종목
statement.receiverBizClass = "수신자 종목";
// 수신자 업태
statement.receiverBizType = "수신자 업태";
// 수신자 담당자 성명
statement.receiverContactName = "수신자 담당자명";
// 수신자 메일주소
statement.receiverEmail = "test@receiver.com";
/**************************************************************************
* 전자명세서 기재항목 *
**************************************************************************/
// [필수] 공급가액 합계
statement.supplyCostTotal = "200000";
// [필수] 세액 합계
statement.taxTotal = "20000";
// 합계금액
statement.totalAmount = "220000";
// 기재상 일련번호 항목
statement.serialNum = "123";
// 기재상 비고 항목
statement.remark1 = "비고1";
statement.remark2 = "비고2";
statement.remark3 = "비고3";
// 사업자등록증 이미지 첨부여부
statement.businessLicenseYN = false;
// 통장사본 이미지 첨부여부
statement.bankBookYN = false;
statement.detailList = new List<StatementDetail>();
StatementDetail detail = new StatementDetail();
detail.serialNum = 1; // 일련번호, 1부터 순차기재, 최대 99
detail.purchaseDT = "20250314"; // 거래일자
detail.itemName = "품목명"; // 품목명
detail.spec = "규격"; // 규격
detail.qty = "1"; // 수량
detail.unitCost = "100000"; // 단가
detail.supplyCost = "100000"; // 공급가액
detail.tax = "10000"; // 세액
detail.remark = "품목비고"; // 비고
detail.spare1 = "spare1"; //여분1
detail.spare1 = "spare2"; //여분2
detail.spare1 = "spare3"; //여분3
detail.spare1 = "spare4"; //여분4
detail.spare1 = "spare5"; //여분5
statement.detailList.Add(detail);
detail = new StatementDetail();
detail.serialNum = 2; // 일련번호, 1부터 순차기재, 최대 99
detail.purchaseDT = "20250314"; // 거래일자
detail.itemName = "품목명"; // 품목명
detail.spec = "규격"; // 규격
detail.qty = "1"; // 수량
detail.unitCost = "100000"; // 단가
detail.supplyCost = "100000"; // 공급가액
detail.tax = "10000"; // 세액
detail.remark = "품목비고"; // 비고
detail.spare1 = "spare1"; //여분1
detail.spare1 = "spare2"; //여분2
detail.spare1 = "spare3"; //여분3
detail.spare1 = "spare4"; //여분4
detail.spare1 = "spare5"; //여분5
statement.detailList.Add(detail);
// 추가속성항목
statement.propertyBag = new propertyBag();
statement.propertyBag.Add("Balance", "15000"); // 전잔액
statement.propertyBag.Add("Deposit", "5000"); // 입금액
statement.propertyBag.Add("CBalance", "20000"); // 현잔액
try
{
Response response = Global.statementService.RegistIssue(testCorpNum, statement, memo, testUserID);
code = response.code.ToString();
message = response.message;
}
catch (PopbillException ex)
{
code = ex.code.ToString();
message = ex.Message;
}
}
}
}
4. 결과 확인
함수 호출 반환 결과는 아래와 같습니다.
- 성공 : Response code 숫자 1 반환
- 실패 : PopbillException 음의 정수 8자리 숫자값 오류코드와 오류메시지 반환 [오류코드]